Arduino Serial Data Logger
Posted By admin On 12.12.20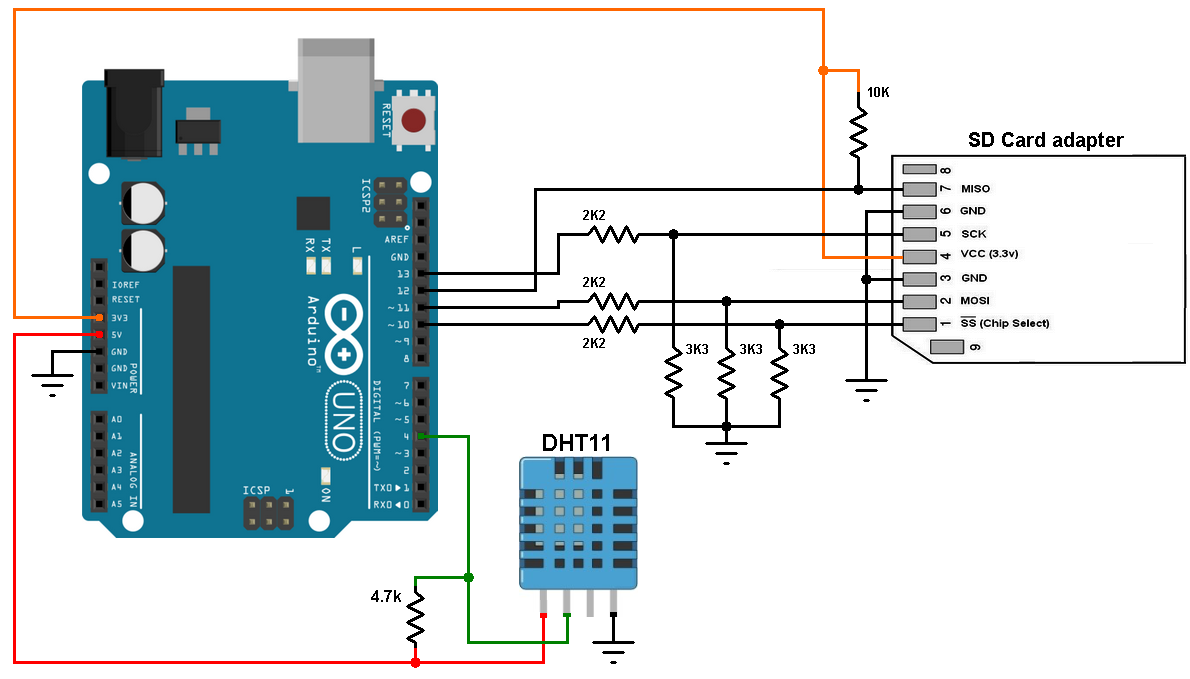
- Data Logging With Arduino
- Arduino Temperature Logger
- Arduino Serial Data Logger Download
- Arduino Serial Data Logger For Mac
- Advanced Serial Data Logger
- Arduino Rs232 Serial Data Logger
- Raspberry Pi Arduino Serial Data Logger
Let's look at the code for this component.
First, look at the third image in this step. It contains some code with two functions:
☀ How to Build an Arduino Data Logger ☀ A data logger is a device that records sensor information at regular intervals over a period of time so that information can be examined later for an understanding of phenomenon that are hard to observe directly. Arduino serial data logger. Want to find out how to build an Arduino data logger and watch data from an Arduino? Here’s a quick way to do this: If you can use an SD card in your project, that is an obvious solution to create an Arduino SD card data logger. Some Ethernet shields are provided with an SD Card reader. ☀ How to Build an Arduino Data Logger ☀ About Us A data logger is a device that records sensor information at regular intervals over a period of time so that information can be examined later for an understanding of phenomenon that are hard to observe directly.
In C++, all functions provide their return type, then the name, then the two round braces that can be used to pass in arguments, usually as variables. In this case, the return type is void, or nothing. The name is setup and the function takes no arguments.
The setup function runs once when Arduino boots (when you plug it in or attach batteries).
Data Logging With Arduino
The loop function runs in a constant loop from the millisecond the setup function completes.
Everything you put in the loop function will run when Arduino runs. Everything outside that will only run when called. Like if we defined and called another function outside the loop.
Task
Open the Code panel with the button in Tinkercad. Change the Blocks dropdown to Text. Agree to the warning box that pops up. Now, delete everything you see except the text in the third image in this step.
Variables
To get started, we need to assign some variables so we make our code really efficient.
Variables are like buckets that can only hold one object (C++ is what we call object-orientated). Yes, we have arrays, but these are special variables and we'll talk about them later. When we assign a variable, we need to tell it what type it is, then give it a value. It looks like this:
So, we assigned a variable and gave it type int. An int is an integer or a whole number.
'But you didn't use a whole number!Real estate empire game. ', I hear you say. That's true.
Arduino does something special for us so we can use A0 as an integer, because in another file it defines A0 as an integer, so we can use the A0 constant to refer to this integer without having to know what it is. If we just typed 0, we would refer to the digital pin at position 0, which wouldn't work.
So, for our code we will write a variable for the sensor we have attached. Whilst I recommend giving it a simple name, that's up to you.
Your code should look like this:
Now, let's tell Arduino how to handle the sensor on that pin. We'll run a function inside setup to set the pin mode and tell Arduino where to look for it.
the pinMode function tells Arduino that the pin (A0) will be used as an INPUT pin. Note the camelCaseUsed (see each first letter is a capital, as in it has humps, hence.. camel..!) for the variables and function names. This is a convention and good to get used to.
Finally, let's use the analogRead function to get some data.
You'll see we stored the reading in a variable. This is important as we need to print it. Let's use the Serial library (a library is code we can add to our code to make things faster for us to write, just by calling it by its definition) to print this to the serial monitor.
A few new things! First, you'll see these:
We use comments to tell other people what our code is doing. You should use these often. The compiler won't read these and convert them in to code.
Now, we also added the Serial library with the line
This is an example of a function that takes an argument. You called the library Serial then ran a function (we know it's a function because of the round braces) and passed in an integer as an argument, setting the Serial function to operate at 9600baud. Don't worry why - just know it works, for now.
The next thing we did was print to the serial monitor. We used two functions:
What's important to see is that each has a separate purpose. Make sure your strings use double-quote marks and that you leave the space after the colon. That helps readability for the user.
Arduino Temperature Logger
Finally, we used the delay function, to slow our loop down and make it only read once every three seconds. This is written in thousands of a second. Change it to read only once every 5 seconds.
Great! On we go!
Now that we’ve got all that downloaded and installed, let’s start with the Arduino part.
Here’s a basic template I created that will display the time in column A and your sensor measurements in column B.
Of course, this is just a basic template, which is pretty straight forward and you can tweak it to suit your needs. Mubarakan songs downloadming.
I’ve added explanations in the Arduino code so you (and I, after not working with it for a while) know which part of the code does what.
Here’s the sketch:
//always starts in line 0 and writes the thing written next to LABEL
void setup() {
Serial.begin(9600); // the bigger number the better
Arduino Serial Data Logger Download
Serial.println('CLEARDATA'); //clears up any data left from previous projects
Serial.println('LABEL,Acolumn,Bcolumn,..'); //always write LABEL, so excel knows the next things will be the names of the columns (instead of Acolumn you could write Time for instance)
Arduino Serial Data Logger For Mac
Serial.println('RESETTIMER'); //resets timer to 0
}
void loop() {
Serial.print('DATA,TIME,TIMER,'); //writes the time in the first column A and the time since the measurements started in column B
Serial.print(Adata);
Serial.print(Bdata);
Serial.println(..); //be sure to add println to the last command so it knows to go into the next row on the second run
Advanced Serial Data Logger
delay(100); //add a delay
Arduino Rs232 Serial Data Logger
}
Obviously if you upload this code, it won’t work on it’s own!
Raspberry Pi Arduino Serial Data Logger
You need to add a formula for Adata, Bdata and … . This template is just for reference so you know how to use the program. Just add Serial.read() function, name it Adata, Bdata and … and it should work.